TextViewにテキストを表示する時に、文字色を部分的に変えたい場合があります。その場合の対処方法を示します。
1.対処例の画面
図1にTextViewに表示するテキストを部分的に文字色を変えた例を示します。
この画面で上側の文字列表示はTextViewに同一色でテキストを表示しました。下側の文字列表示は別のTextViewに部分的に色を変えてテキストを表示しました。
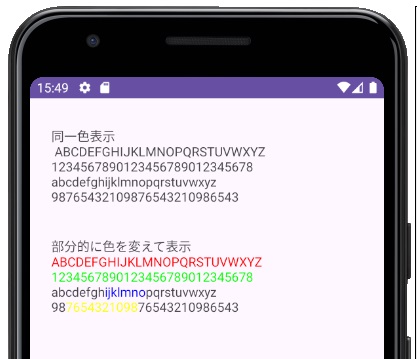
2.開発環境等
開発ツールバージョン: Android Studio Giraffe 2022.3.1 Path3
使用言語: Kotlin
3.対処方法
色を変えたい文字列部分を<font color–>と</color>のタグで挟み、それをString型からSpanned型に変換してTextViewにセットします。
具体的にはテキストの色を変えたい文字列部分を
<font color=”色名称”>と</font>で挟みます。
(例)
val text = “ABCDEFGHI\r\n”
の”CDEF”のみ赤字としたい場合は
val text = “AB<font color=\”red\”>CDEF</font>GHI<br>”
とします。
元のテキストの”\r\n”は改行ですが、代わりに<br>タグに変更します。
このString型のtextをHtml.fromHtml()メソッドを使用して、マークアップオブジェクトが添付されているテキストであるSpanned型に変換して、対象となるTextViewにsetText()メソッドでセットします。
4.対処例のプログラム
4-1.画面レイアウトのXMLコード
レイアウト(app→res→layout→activity_main.xml)のXMLコードを以下に示します。上下に二つのTextViewを配置して、上のTextViewのidをtextView1、下のTextViewのidをtextView2にしました。
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView1"
android:layout_width="363dp"
android:layout_height="90dp"
android:layout_marginStart="24dp"
android:layout_marginTop="32dp"
android:text="TextView"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView2"
android:layout_width="362dp"
android:layout_height="90dp"
android:layout_marginStart="24dp"
android:layout_marginTop="32dp"
android:text="TextView"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView1" />
</androidx.constraintlayout.widget.ConstraintLayout>
4-2.Kotlinプログラムコード
Kotlin (MainActivity.kt)のプログラムコードを以下に示します。
7行目~14行目:
図1の画面でテキストの文字色が全て同じ色で表示されている上側のTextView表示部分のコードで、特に何もせずにString型のテキストをTextViewにセットしています。文字列の末尾に”\r\n”を付けて改行しています。
17行目~24行目
図1の画面でテキストの文字色が部分的に異なった色で表示されている下側のTextView表示部分のコードです。
テキストの色を変えたい部分を<font color=”色名称”>と</font>で挟んでいます。
また、文字列の末尾の”\r\n”は<br>に変更しています。
そのテキストをString型からHtml.fromHtml()メソッド(24行目)を使用してマークアップオブジェクトが添付されているテキストスタイルであるSpanned型に変換して、TextViewにsetText()メソッドを使用してセットしています。
Html.fromHtml()メソッドの引数 html.FROM_HTML_MODE_LEGACYは、古いAndroidバージョンとの互換性を重視して空白や改行をそのまま保持するモードを指定しています。
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
//------単一色でTextViewに表示---------------------
val text1: String = "同一色表示\n\r" +
"ABCDEFGHIJKLMNOPQRSTUVWXYZ\r\n" +
"1234567890123456789012345678\r\n" +
"abcdefghijklmnopqrstuvwxyz\r\n" +
"98765432109876543210986543\r\n"
val tView1 = findViewById<TextView>(R.id.textView1)
tView1.text = text1
//------部分的に色を変えてTextViewに表示------------
val text2: String = "部分的に色を変えて表示<br>" +
"<font color=\"red\">ABCDEFGHIJKLMNOPQRSTUVWXYZ</font><br>" +
"<font color=\"green\">1234567890123456789012345678</font><br>" +
"abcdefgh<font color=\"blue\">ijklmno</font>pqrstuvwxyz<br>" +
"98<font color=\"yellow\">7654321098</font>76543210986543<br>"
val tView2 = findViewById<TextView>(R.id.textView2)
tView2.setText(Html.fromHtml(text2, Html.FROM_HTML_MODE_LEGACY))
}
}